N3MIS15
Well-Known Member
- Joined
- 4/10/10
- Messages
- 97
- Reaction score
- 41
I was hesitant to post this as the code is very bare bones, has absolutely zero error handling and probably totally the wrong way to go about this. I did however get working exactly what i was after.
For $18~ on aliexpress and a bit of dodgy code I can now check my tilt hydrometer readings at a glance.
Here is the Arduino "code" if anyone cares.
It takes the first bluetooth device it finds and attempts to pull tilt data from it. If it succeeds, the device sleeps for 1 hour before scanning again. If it does not succeed the device keeps scanning until it does.
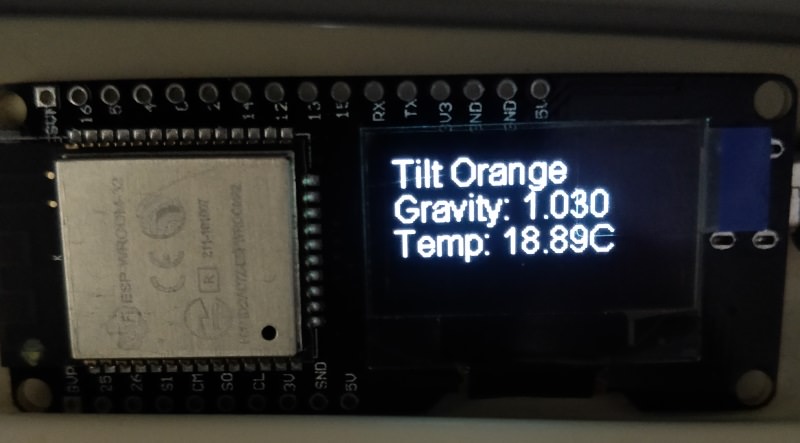
For $18~ on aliexpress and a bit of dodgy code I can now check my tilt hydrometer readings at a glance.
Here is the Arduino "code" if anyone cares.
Code:
#include <stdlib.h>
#include <BLEDevice.h>
#include <BLEUtils.h>
#include <BLEScan.h>
#include "SSD1306.h" // https://github.com/ThingPulse/esp8266-oled-ssd1306
SSD1306 display(0x3c, 5, 4);
int scanTime = 5; //In seconds
void setup() {
display.init();
display.flipScreenVertically();
display.clear();
}
void loop() {
display.clear();
display.setTextAlignment(TEXT_ALIGN_LEFT);
display.setFont(ArialMT_Plain_16);
display.drawString(0, 0, "Scanning..."); // Line 1
display.display();
BLEDevice::init("");
BLEScan* pBLEScan = BLEDevice::getScan(); //create new scan
BLEScanResults foundDevices = pBLEScan->start(scanTime);
BLEAdvertisedDevice tilt = foundDevices.getDevice(0);
char *pHex = BLEUtils::buildHexData(nullptr, (uint8_t*)tilt.getManufacturerData().data(), tilt.getManufacturerData().length());
String manufacturerData = pHex;
String UUID = manufacturerData.substring(8, 40);
const char* xTemp = (manufacturerData.substring(40, 42) + manufacturerData.substring(42, 44)).c_str();
long tempF = strtol(xTemp, NULL, 16);
float tempC = (float(tempF-32)*0.55555555555555555555555555555556);
const char* xGravity = (manufacturerData.substring(44, 46) + manufacturerData.substring(46, 48)).c_str();
long gravityThousand = strtol(xGravity, NULL, 16);
// Lets get the colour, because why not.
String tiltColour = "";
if(UUID.substring(6, 7).equals("1")) {
tiltColour = "Red";
}
else if (UUID.substring(6, 7).equals("2")) {
tiltColour = "Green";
}
else if (UUID.substring(6, 7).equals("3")) {
tiltColour = "Black";
}
else if (UUID.substring(6, 7).equals("4")) {
tiltColour = "Purple";
}
else if (UUID.substring(6, 7).equals("5")) {
tiltColour = "Orange";
}
else if (UUID.substring(6, 7).equals("6")) {
tiltColour = "Blue";
}
else if (UUID.substring(6, 7).equals("7")) {
tiltColour = "Yellow";
}
else if (UUID.substring(6, 7).equals("8")) {
tiltColour = "Pink";
}
display.clear();
display.drawString(0, 0, "Tilt " + tiltColour); // Line 1
display.drawString(0, 16, "Gravity: " + String(float(gravityThousand)/1000, 3)); // Line 2
display.drawString(0, 32, "Temp: " + String(tempC) + "C"); // Line 3
//display.drawString(0, 48, "Line 4"); // Line 4
display.display();
delay(3600000);
}
It takes the first bluetooth device it finds and attempts to pull tilt data from it. If it succeeds, the device sleeps for 1 hour before scanning again. If it does not succeed the device keeps scanning until it does.